Android Application Development
Introduction: It is a short term course of six months. This course is for the Engineering (B.Tech(IT/CS) /MCA/BCA/B.Sc (IT/CS) students for their Industrial Training Programme/Project Work. Students of NIELIT ‘A’/’B’ Levels can also take admission in this course and take an entry to the Software Industry as an Android App Developer. The syllabus of the course is very much parallel with the requirements of modern job demands in software industry.
Eligibility:
(1) KNOWLEDGE OF ANY PROGRAMMING LANGUAGE (C++/JAVA etc.)
(2) BASIC KNOWLEDGE OF DBMS.
Block Syllabus:
1) Introduction to Mobile Apps
- Why we Need Mobile Apps
- Different Kinds of Mobile Apps
- Briefly about Android
2) Introduction Android
- History Behind Android Development
- What is Android?
- Pre-requisites to learn Android
- Brief Discussion on Java Programming
3) Android Architecture
- Overview of Android Stack
- Android Features
- Introduction to OS layers
4) Deep Overview in Android Stack
- Linux Kernel
- Libraries
- Android Runtime
- Application Framework
- Dalvik VM
5) Installing Android Machine
- Setting up Android Studio
- Working with Android Studio
6) Creating First Android Application
- Creating Android Project
- Debugging Application through DDMS
- Setting up environment
- AVD Creation
- Executing Project on Android Screen
7) Android Components
- Activities
- Services
- Broadcast Receivers
- Content Providers
8) Hello World App
- Creating your first project
- The manifest file
- Layout resource
- Running your app on Emulator
9) Building UI with Activities
- Activities
- Views, layouts and Common UI components
- Creating UI through code and XML
- Activity lifecycle
- Intents
- Communicating data among Activities
10) Advanced UI
- Selection components (GridView, ListView, Spinner )
- Adapters, Custom Adapters
- Complex UI components
Building UI for performance - Menus
- Creating custom and compound Views
11) Notifications
- Toast, Custom Toast
- Dialogs
- Status bar Notifications
12) Multithreading
- Using Java Mutithreading classes
- AsyncTask
- Handler
- Post
13) Styles And Themes
- Creating and Applying simple Style
- Inheriting built-in Style and User defined style
- Using Styles as themes
14) Resources and Assets
- Android Resource
- Using resources in XML and code
- Localization
- Handling Runtime configuration changes
15) Intent, Intent Filters and Broadcast Receivers
- Role of filters
- Intent-matching rules
- Filters in your manifest
- Filters in dynamic Broadcast Receivers
- Creating Broadcast receiver
Receiving System Broadcast
- Understanding Broadcast action, category and data
- Registering Broadcast receiver through code and through XML
- Sending Broadcast
16) Data Storage
- Shared Preferences
- Android File System
- Internal storage
- External storage
- SQLite
17) IntroducingSQLite
- SQLiteOpenHelper and creating a database
- Opening and closing adatabase
- Working with cursors Inserts, updates, and deletes
- Network
17) Content Providers
- Accessing built in content providers
- Content provider MIME types
- Searching for content
- Adding, changing, and removing content
- Creating content provider
- Working with content files
18)Services
- Overview of services in Android
- Implementing a Service
- Service lifecycle
- Inter Process Communication (AIDL Services)
19) Multimedia in Android
- Multimedia Supported audio formats
- Simple media playback
- Supported video formats
- Simple video playback
20) Location Based Services and Google Maps
- Using Location Based Services
- Finding current location and listening for changes in location
- Proximity alerts
- Working with Google Maps
- Showing google map in an Activity
- Map Overlays
- Itemized overlays
- Geocoder
- Displaying route on map
21) Web Services and WebView
- Consuming web services
- Receiving HTTP Response (XML, JSON )
- Parsing JSON and XML
- Using WebView
22) Sensors
- How Sensors work
- Using Orientation and Accelerometer sensors
- Best practices for performance
23) WiFi
- Monitoring and managing Internet connectivity
- Managing active connections
- Managing WiFi networks
24) Telephony Services
- Making calls
- Monitoring data connectivity and activity
- Accessing phone properties and status
- Controlling the phone
- Sending messages
25) Camera
- Taking pictures
- Media Recorder
- Rendering previews
26) Bluetooth
- Controlling local Bluetooth device
- Discovering and bonding with Bluetooth devices
- Managing Bluetooth connections
- Communicating with Bluetooth
26) More
- Fragments
- Material Design
- Gradle and Permissions for new versions
- NEW TOPICS: Since each new version of Android has new features like FCM (FIREBASE CLOUD MESSAGING), we keep extra time for adding custom topics in every batch. You can request any Android Topic.
Project Work & Certificate: After completion of the training programme, we shall involve the students on a Project Work where they can get an experience of handing live projects of software industry. After submission of the Project Work the students will get a Certificate of the Institute.
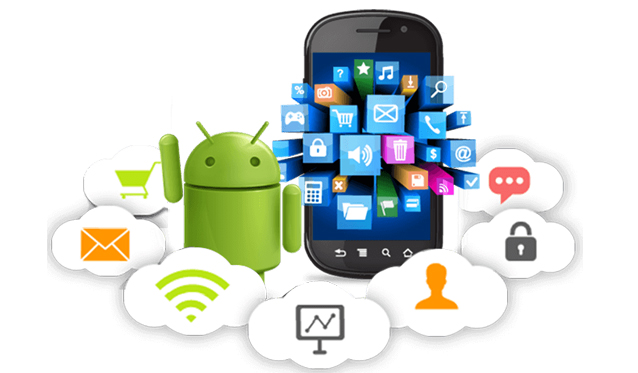